function toggleStylesheet(href,onoff){
var existingNode=0 //get existing node:
for(var i = 0; i < document.styleSheets.length; i++){
if( document.styleSheets[i].href && document.styleSheets[i].href.indexOf(href)>-1 ) existingNode = document.styleSheets[i].ownerNode
}
if(onoff == undefined) onoff = !existingNode //toggle on or off if undefined
if(onoff){ //TURN ON:
if(existingNode) return onoff
var link = document.createElement('link');
link.rel = 'stylesheet';
link.type = 'text/css';
link.href = href;
document.getElementsByTagName('head')[0].appendChild(link);
}else{ //TURN OFF:
if(existingNode) existingNode.parentNode.removeChild(existingNode)
}
return onoff
}
//EXAMPLES:
toggleStylesheet('myStyle.css') //toggle myStyle.css on or off
toggleStylesheet('myStyle.css',1) //add myStyle.css
toggleStylesheet('myStyle.css',0) //remove myStyle.css
Cron's Web Tech Blog
Useful scripts, utilities and classes for developers of HTML, PHP, Javascript, Actionscript, mySQL, CSS, etc. This blog will fill the occasional gaps in information I stumble across in my daily developing. If there's something I feel is missing from the vast pool of stuff already out there, I'll post it here. I'm a nice guy. You'd like me.
crondesign.com website design blog
08 March 2016
Add or remove a CSS stylesheet to HTML using plain vanilla Javascript
This little function will attach or delete a new stylesheet to your html page using good old JS. The second parameter is optional so it will toggle on or off the stylesheet, depending on it's current setting. The function will return true or false (1 or 0), depending on weather the style is on or off. Enjoy!
10 December 2015
Repeat a string in PHP with iteration counter - quick and easy function
When you're working through some complex program logic, low level code like manipulating strings is a distraction to write and worse, when you come back to it six months later, it makes your code look long and daunting to get back into. With this in mind, I always try to wrap low level code in re-usable functions.
Here's one such handy little function which works just like PHP's native str_repeat but it will include the iteration count in the string itself and do some cleaning at the end. This is perfect for generating numbered lists, sql queries and a bunch of other uses.
Continue Reading >
Here's one such handy little function which works just like PHP's native str_repeat but it will include the iteration count in the string itself and do some cleaning at the end. This is perfect for generating numbered lists, sql queries and a bunch of other uses.
06 February 2014
35% off GoDaddy Discount code voucher coupon - Referral code
WOWCWTB
Just enter the code during checkout
This voucher code coupon will get you a 35% discount off GoDaddy.com products. This discount code should not expire as because it's not a voucher or coupon code - it's a referral code.
...
Labels:
tips
21 September 2012
Loop Javascript (or Actionscript!) array based on index
Looping arrays is particularly useful for things like photo galleries and other iterative processes where you want the "next photo" button on a gallery to continue from the beginning of the album after the user passes the last photo.
In my humble opinion, I believe this functionality should be built into the core of Ecmascript ( For example, something like myArray[-2] should really return the second last element in the array) but alas we have to write it ourselves, so here goes...
Continue Reading >
In my humble opinion, I believe this functionality should be built into the core of Ecmascript ( For example, something like myArray[-2] should really return the second last element in the array) but alas we have to write it ourselves, so here goes...
Labels:
Actionscript,
AS2,
AS3,
functions,
Javascript,
js
28 May 2012
Add Photoshop PSD & Illustrator AI folder thumbnails to all versions of Windows XP/2003/Vista/2008/7 32/64-bit
This is great. Adobe have never bothered to provide thumbnail support for windows machines. I dont know if this is because they are technically incompetent, lazy or they are just trying to promote their bloated Adobe Bridge viewer application. Either way, as you can probably tell from the tone, it bugs me. Users have been screaming for this no-brainer feature since photoshop first arrived on the windows platform in 1992. Yes that's 20 years ago. Anyway, I've been applying registry hacks and messing with dlls for years to get thumbnails going in windows explorer but I finally found this tool today which is a quick and simple solution that claims to work across all versions of both windows and Adobe CS.
The installer below makes windows automatically load thumbs for all versions of Photoshop and Illustrator in the normal windows folder view.
Continue Reading >
The installer below makes windows automatically load thumbs for all versions of Photoshop and Illustrator in the normal windows folder view.
11 May 2012
Simple Javascript Pie Chart using HTML5 Canvas tag
I had a look around today for a handy pie chart that I could quickly drop into a new web app I'm working on and it seems there's a lot of bloated solutions out there. I like to keep things short and sweet so I found this one online and edited it to make it a bit more user friendly. Enjoy!
Labels:
functions,
html,
Javascript,
js
23 March 2012
AS3 Load external flash SWFs into a queue to play in a consecutive sequence
This script imports a text file from the local directory and imports all swfs listed in the text file to play on the stage sequentially, i.e. one after another. Its very useful for banner ads and other animations when you need to be able to slot new ads into the sequence.
Continue Reading >
Labels:
Actionscript,
AS3
26 January 2012
Free Javascript for Rotating Website Banner or Fading Slideshow - standalone
As flash becomes less widespread I've had more and more instances where I need to create website banners in Javascript rather than my old flash approach. Today I've decided to share my method with my adoring public (that's you!).
This method is completely standalone and does not require jQuery or any other plugin or library. It has been tested and works in latest versions of all browsers.
View a demo here (right click to get the whole source at once) or view the step-by-step instructions below...
Continue Reading >
This method is completely standalone and does not require jQuery or any other plugin or library. It has been tested and works in latest versions of all browsers.
View a demo here (right click to get the whole source at once) or view the step-by-step instructions below...
Labels:
functions,
Javascript,
js
13 November 2011
Print CSS backgrounds in Google Chrome & Safari webkit
Up until now it's been impossible, but a new fix published this week by the chrome team makes it simple for a site developer to trigger background printing using CSS...
Continue Reading >
05 November 2011
Javascript function for checking a variables type or empty/unset variables - similar to PHP's empty()
I've been building on this one all week and I'm finding it more and more useful for quickly writing variable checks at the top of functions. You can use it to easily check if a var is defined or check if it is of a certain type. It's a work in progress but it's very handy. Check out the commented examples to get an idea of potential uses. Ideas for improvements/additions are welcome!
Continue Reading >
Labels:
functions,
Javascript,
js
12 October 2011
How to repair Google Apps setup server errors
I got a tip today from a friend of mine working in Google. A server error occurred during the registration of my new domain and I was left with no gApps and no tech support (anyone on gApps standard will notice there is absolutely no way to contact anyone remotely related to a google employee).
Continue Reading >
Anyway it seems the most common of these server errors is that the account you register with is not given admin rights. Here's the fix...
Labels:
tips
19 July 2011
Most simple/basic single level CSS dropdown menu without Javascript tutorial
Sometimes when web demos focus on extended functionality, it's difficult to identify and extract exactly what you need for your own project from the examples. With beginners & newcomers to CSS in mind, I've put together this extremely simple dropdown menu & tutorial. You can take this skeleton and add styling and content for your own project. This menu auto sizes/auto expands to fit its content and could easily be adapted to add more levels, animation or fancy styling.
Check out the demo here
Continue Reading >
Check out the demo here
17 July 2011
AS3: Simple, Powerful String Find & Replace all instances function for Flash Actionscript. Similar to PHP's str_replace replacement.
Continue Reading >
Labels:
Actionscript,
AS3,
functions,
shortcuts
AS3: Wave of values class function - produces incremental and decremental values
Sometimes it's necessary to have a value increment until it gets to a certain point and then have it decrement again in a wave shape. A simple Math.sin() calculation will work in some cases but its not easy to control the increment using that method. This handy & simple class makes it quick and easy to do just this. There's lots of room for improvements & additions so feel free to post updates :)
Continue Reading >
Labels:
Actionscript,
AS3,
functions
05 June 2011
AS3 handy weighted random & rounding functions with decimal/floating point precision
Like a lot of the "improvements" Adobe made to Actionscript with AS3, the Math.random() function is now a pain in the ass. It only generates a floating point number between 0 and 1 so 99% of the time, you need a few lines of code to get your random on.
With time saving in mind, I've written this weighted random function which makes beautiful randomness a lot handier to implement. For best results throw this in a class package and include it in every project.
Here it comes...
Continue Reading >
With time saving in mind, I've written this weighted random function which makes beautiful randomness a lot handier to implement. For best results throw this in a class package and include it in every project.
Here it comes...
Labels:
Actionscript,
AS3,
functions,
shortcuts
23 April 2011
SugarSync referral link - Better than dropbox
If you dont backup your computer already, try this... It automatically keeps your documents synced to the web in case your computer dies someday. Its better than dropbox because you can choose any folders on your computer to backup and you get more space. You can also share any backed up file with friends/clients with a simple right click. You get 5.5GB free with this referral link (I get an extra bonus too so everyone's a winner). For the less tech savvy of you: just install it and it will ask you what folders on your computer are important. After that you can forget about it until your computer dies.
Here's the link: (for 5.5GB free cloud storage)
>> SugarSync referral
Here's the link: (for 5.5GB free cloud storage)
>> SugarSync referral
Labels:
tips
18 April 2011
AS3 function to rotate a 2D or 3D MovieClip or Sprite around its center or change to any registration point
OK here we go - I looked everywhere for this one but only found bits of code from various forums and blogs that were part of classes and packages and as such, full of dependent variables. Anyway, after a bit of advice from the very helpful community at StackOverflow and a bit of tinkering, she's up and running!
The function below will rotate a DisplayObject around any point on a 2D or 3D plane. This is particularly useful for rotating around an object's center point so this is the default behaviour. Enjoy!
Continue Reading >
The function below will rotate a DisplayObject around any point on a 2D or 3D plane. This is particularly useful for rotating around an object's center point so this is the default behaviour. Enjoy!
Labels:
Actionscript,
AS3,
functions
14 March 2011
Max/MSP Javascript "find object" - lazy dollar function
The second function in my "Making Max JS less crap" series, this function scans all objects in the patch and all subpatches and returns a reference to the object with the specified scripting name: It's basically a lazy man's version of my dollar function which means you dont need to give every patcher a scripting name. The downside is it (probably) runs a bit slower. Enjoy!
Continue Reading >
Labels:
functions,
Javascript,
js,
Max,
Max/MSP
Max/MSP Javascript: Dollar Function - Returns a reference to a Max Object in a patch/subpatch
I started using Max this month and was simultaneously impressed with its power and simplicity and frustrated by it's cumbersome interface. I quickly checked out it's javascript capabilities and became much more comfortable. JS allows you do simple operations quite quickly that would take a tonne of parsing objects which use up space and lead to a messy patch.
That said, JS in Max is really a slightly ugly cousin of browser based javascript. Calls to objects are long winded and basically everything is a bit head wrecking!
So here we go: the first in my "Making Max JS less crap" series... The infamous dollar function! All you need to do to access a max object is give the object a scripting name in the inspector* and then use: $('scriptingname')
Continue Reading >
That said, JS in Max is really a slightly ugly cousin of browser based javascript. Calls to objects are long winded and basically everything is a bit head wrecking!
So here we go: the first in my "Making Max JS less crap" series... The infamous dollar function! All you need to do to access a max object is give the object a scripting name in the inspector* and then use: $('scriptingname')
Labels:
functions,
Javascript,
js,
Max,
Max/MSP
13 February 2011
Actionscript 3: Better Random Function & Better Rounding Function
I was amazed at how lame the rounding and random functions are in AS3 so I've written 2 simple functions to add much needed functionality. Enjoy...
Continue Reading >
Labels:
Actionscript,
AS3,
functions
11 February 2011
Actionscript/Javascript Colour mode conversion functions - convert HSB/HSL/HSV to RGB to web HEX color or to flash matrix
I wrote/found/tweaked these conversion functions today to convert between the most common colour modes. it was very difficult to find a solid source for converting colours online so I thought I'd post them here for the world. They should work in actionscript 2 & 3 and could easily be tweaked to work in php, python or any other language. Here goes...
Continue Reading >
Labels:
Actionscript,
AS2,
AS3,
functions,
Javascript,
js
23 August 2010
Javascript: Simple, Drag & Drop element class - multiple draggable elements on one page.
I found a tonne of these drag & drop functions online but they were all either too big & bloated or relied heavily on external libraries. The one I did find that was small and usable triggers chrome bug 7423 because it captures the mousedown event for the page. So I've written my own and posted here for your convenience.
See Demo (Internet Explorer ain't supported)
Continue Reading >
See Demo (Internet Explorer ain't supported)
Labels:
functions,
Javascript,
js
29 June 2010
AS2: Simple, Powerful String Find & Replace all instances function for Flash Actionscript. Similar to PHP's str_replace replacement.
Continue Reading >
Labels:
Actionscript,
AS2,
functions
18 June 2010
Resize a Movie Clip containing a mask by getting dimensions of visible bounds/area and compensating width/height
This was a head wrecker and took me most of the day today. Actionscript does not see Movie Clips as we do and considers invisible content to be part of the MC. As such, when you try to resize an MC that contains masked content which extends beyond what we consider to be the edges of the MovieClip, flash makes a balls of it.
To counteract this I've written and rewritten a double function which resizes your clip based on the visible area rather than what flash considers the bounds. Here it comes...
Continue Reading >
To counteract this I've written and rewritten a double function which resizes your clip based on the visible area rather than what flash considers the bounds. Here it comes...
Labels:
Actionscript,
AS2,
functions
13 May 2010
Universal ALL DOMAIN rewrite for non-www to canonical/www using htaccess or https.conf on apache server
This took me a few days to get right. The lack of feedback from testing, along with browser caching and the general head wrecking-ness of Regex make htaccess rewrites like this a total pain in the ass. Anyway thanks to the guys over at the alt.apache.configuration newsgroup, I've managed this:
The code below will rewrite anything on your server that does not have a www. at the beginning to the same thing with a www. prefixed. Urls beginning with an IP address are left alone so that http://IPADDRESS/~username/ access still works.
Subdomains are also redirected to their www. equivalent however they should still resolve and work normally. (untested)
This is great if you run your own server and want to redirect everything at once or if you just want a template that you can use for all your websites without editing or altering.
My code works without too much Regex with makes it a lot less memory intensive than most "universal" mod_rewrites out there.
06 May 2010
PHP: Ultimate get/fetch RELATIVE PATH function between 2 folders/directories/files/pages or root dir
I wrote these functions today when everything I found online that attempted the same job was either too bulky, or too restrictive. It took me flippin ages - far longer than it should have! But it has huge functionality and will be a great addition to the arsenal.
The main function relpath basically calculates the relative path from anywhere to anywhere on the same domain. Possible uses are listed below:
Continue Reading >
- Get relative path from the current page to the root directory of the local domain (rootpath)...
echo relpath(); //outputs ../../ or something similar
- Get relative path from the current page to any other file/folder on the domain...
echo relpath("/scripts/javascripts/jsmin/min.js"); //outputs ../../jsmin/min.js
- Get relative path from any file/folder on the domain to any other file/folder on the domain...
echo relpath("/scripts/connect.php","/scripts/javascripts/jsmin"); //outputs ../../scripts.connect.php
02 May 2010
PHP: Quick & handy browser sniffer/checker function/class
Yes, you can check your visitor's browser using PHP! What's more, it never goes out of date! It automatically updates to recognise the lastest browsers. As much as I'd like to, I can't take credit for this one - it's the work of Jonathan Stoppani and makes browser sniffing with PHP an absolute breeze.
This class (named Browscap) is a great alternative to PHP's native get_broswer function which requires manual regular updating of a browser.ini definitions file. Instead, Browscap intermittently imports the latest browser definitions from browserproject.com so that your scripts are always up to speed with the latest browser information.
Instructions and download below...
Continue Reading >
This class (named Browscap) is a great alternative to PHP's native get_broswer function which requires manual regular updating of a browser.ini definitions file. Instead, Browscap intermittently imports the latest browser definitions from browserproject.com so that your scripts are always up to speed with the latest browser information.
Instructions and download below...
Javascript: Auto-Expand a form's select box on hover/mouseover
Productivity is all about cutting down mouse clicks. With this in mind, I've written this function to open/close a html dropdown menu on mouseover/out. It's currently more of an idea than a usable function, so it might need some testing & tweaking to work cross browser in a real web environment but the basic principal is here to get you started.
Try a demo »
Continue Reading >
Try a demo »
Labels:
css,
html,
Javascript,
js,
usability
28 April 2010
Javascript: Set or get URL/URI/src/href of an iframe or frame/frameset function
This function simply targets an iframe or frameset and retrieves or changes it's src or href attribute.
Continue Reading >
Labels:
Javascript,
js,
shortcuts
27 April 2010
Add padding or a solid background to a Movie Clip with Flash Actionscript AS2
I wrote this little function today to add HTML style padding to a Flash MovieClip. It took a lot of testing to get working but it's actually very straight forward and quite a handy one to have in your function arsenal.
It basically draws a rectangle behind all content in the movie clip and repositions the clip on the Stage. It can also be used to add a solid background behind a given MovieClip. Implementation is a synch:
Continue Reading >
It basically draws a rectangle behind all content in the movie clip and repositions the clip on the Stage. It can also be used to add a solid background behind a given MovieClip. Implementation is a synch:
Labels:
Actionscript,
AS2
21 April 2010
PHP: Image resize function for calculating & constraining image proportions to fit in a box or space
I wrote this function on the back of my Actionscript version to calculate the output size of an image. If you only have a certain amount of space available in your page layout and you need to dynamically display an image that you don't know the size of, then this function is for you.
You simply specify how much space you have and the function will calculate the new size of the image proportionately and (optionally) return the html img tag for the browser. Sounds simple but it's incredibly useful and a lot easier than coding this each time you have need.
Continue Reading >
You simply specify how much space you have and the function will calculate the new size of the image proportionately and (optionally) return the html img tag for the browser. Sounds simple but it's incredibly useful and a lot easier than coding this each time you have need.
27 November 2009
PHP: How to Compare Directory Attributes & all files within
I wrote this function today to compare the contents of two folders or directories based on 3 comparison points:
This function returns a 3D array containing a list of all differences between the contents of the two directories...
Continue Reading >
- Do files exist in both directories?
- Were they both modified at the same time?
- Are the contents identical?
This function returns a 3D array containing a list of all differences between the contents of the two directories...
25 November 2009
Javascript: Get/Set Caret Cursor position in iframe WYSIWYG editor
I've been working on this for 3 months now on and off, using code snippets from the net and asking questions in forums, etc. I'm trying to get a RTE to autosave as the user is typing. I still don't have a viable solution that works cross browser but I will keep this post updated as I progress. The following works in Firefox:
Continue Reading >
Labels:
Javascript,
js
24 November 2009
PHP: How to upload ZIP, check if file exists within it & extract to the server
I had to do this today for a job. It took far longer than it should have. The resources online are minimal and php.net does not mention any keywords like file_exists on manual for the function needed to do this. so...
Here is the best way to check if an uploaded zip archive contains a certain file and if so, extract the entire contents to a directory on your server:
Continue Reading >
Here is the best way to check if an uploaded zip archive contains a certain file and if so, extract the entire contents to a directory on your server:
11 November 2009
AS2: PHP style trim for Actionscript 2 - with 'any' character support
Theres a few of these functions around but none (that I could find) will allow you to trim non whitespace characters so I pulled this one together which lets you trim any character you want.
//USAGE:
trace(trim(";A test;;", ";")); //outputs: "A test"
function trim(str:String, char:String):String{
if(char==undefined){char=" ";}
code=char.charCodeAt(0)
for(var i = 0; str.charCodeAt(i) ==code; i++);
for(var j = str.length-1; str.charCodeAt(j) ==code; j--);
return str.substring(i, j+1);
}
Labels:
Actionscript,
AS2,
php
09 November 2009
Free AS2 Script for Rotating Website Banner or Slideshow in Flash
Full instructions and demo below...
Labels:
Actionscript,
AS2
19 October 2009
Resize function for image or MovieClip with advanced options - Actionscript2 AS2
I found myself often needing to resize images to fit different spaces using actionscript so I wrote this handy function a while back. It allows you to quickly resize the image proportionally to fit your needs and doesn't require much thought. See usage examples below and you'll see what I mean.
Continue Reading >
Labels:
Actionscript,
AS2,
shortcuts
14 October 2009
mySQL to Flash import class - run queries from AS2 simply & automatically
mySQL2AS package with DataGrid...
This incredibly simple tool takes 5 minutes to set up and allows you to run mySQL queries and return results to flash using Actionscript 2 just as you can in PHP or any other language. Just 3 lines at the top of your flash document make mySQL queries a single function call away. You can loop through the results, trace them all to the output panel and even display them in a datagrid (a flash table) on the stage.
Continue Reading >
This incredibly simple tool takes 5 minutes to set up and allows you to run mySQL queries and return results to flash using Actionscript 2 just as you can in PHP or any other language. Just 3 lines at the top of your flash document make mySQL queries a single function call away. You can loop through the results, trace them all to the output panel and even display them in a datagrid (a flash table) on the stage.
Labels:
Actionscript,
AS2,
php,
shortcuts
04 October 2009
Save JPG /PNG image from AS2 project using AS3 & PHP
There's a tonne of Actionscript 2 image capture scripts out there but after an extensive search last month, the best ones I could find all ran into horrible memory problems and server timeouts when trying to capture large images. So I figured out a better way...
Continue Reading >
Labels:
Actionscript,
AS2,
AS3,
php
26 September 2009
Javascript ScrollFix: Remember scroll position after page reloads.
I've seen a tonne of great web apps with this annoying quirk: When you press save or OK on a settings page, it jumps back to the top as the page reloads. Even some high profile products like Gmail have this irritating usability issue. (Try merging 2 contacts at the bottom of your list and watch the list jump back to the top)
This little bit of javascript saves a cookie when the page is unloaded and attempts to read it and set the scroll position the next time the page is loaded.
Continue Reading >
This little bit of javascript saves a cookie when the page is unloaded and attempts to read it and set the scroll position the next time the page is loaded.
Labels:
cookie,
html,
Javascript,
usability
18 September 2009
PHP class to Import/Export mySQL to CSV or Excel
Import/Export mySQL to CSV class:
This class (not really a class but thought the keyword "class" might help people searching for stuff like this) contains a function which can be fed a mySQL query and a filename. The query is run and the results are saved to a CSV file. There is also a function to import the CSV file back into a database.
This class (not really a class but thought the keyword "class" might help people searching for stuff like this) contains a function which can be fed a mySQL query and a filename. The query is run and the results are saved to a CSV file. There is also a function to import the CSV file back into a database.
30 August 2009
About the banner...
The banner image for this blog was my my first attempt at generating something pretty using Flash Actionscript. I later did a few more experiments and generated the image in the background of this blog too.
View banner image full size:
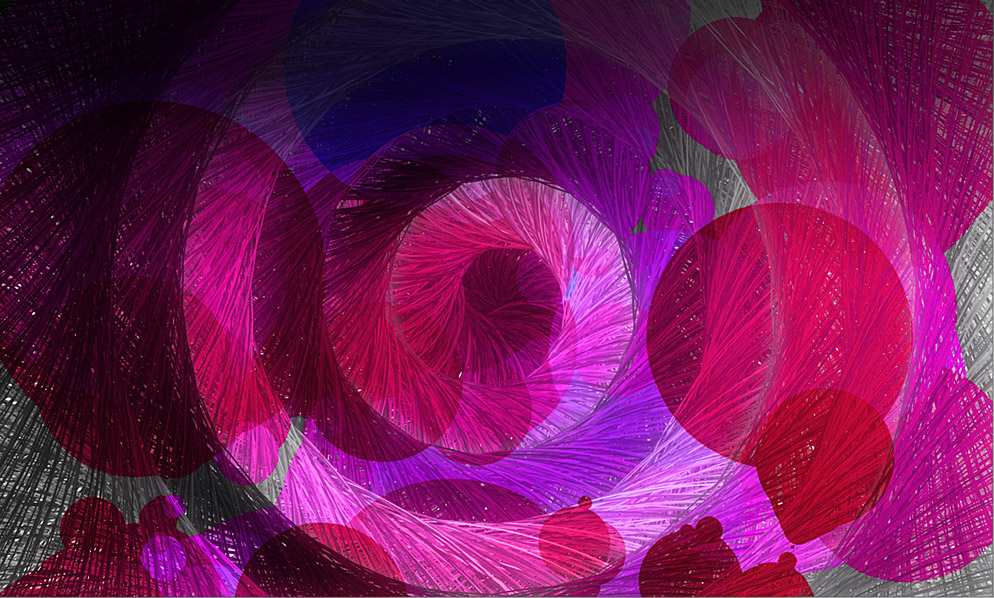
View banner image full size:
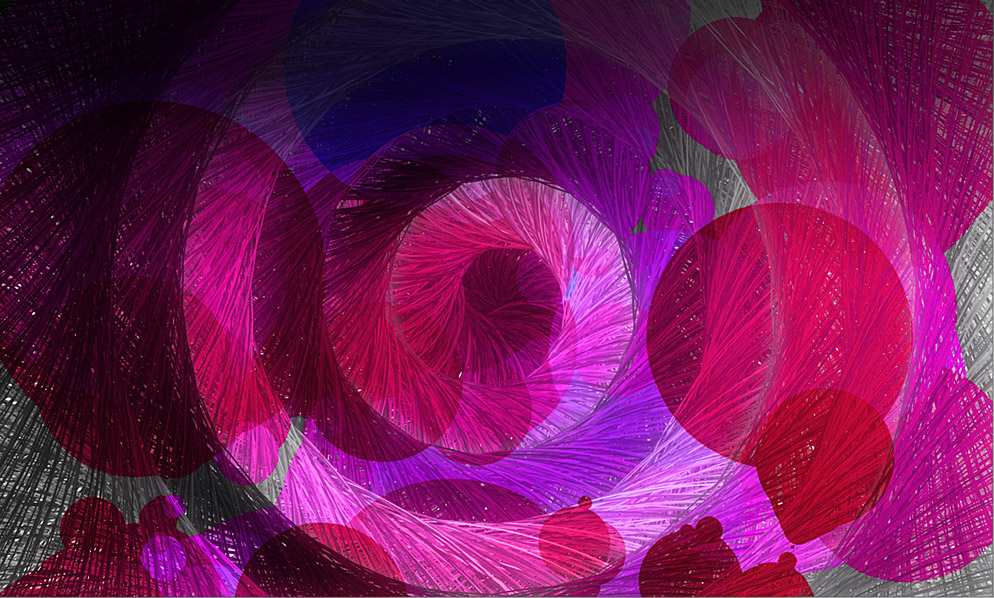
Labels:
Actionscript,
Generative Art
29 August 2009
Hello World!
A new blog: This blog will fill the occasional gaps in information I stumble across in my daily developing. If there's something I feel is missing from the vast pool of stuff already out there, I'll post it here.
I'm a nice guy. You'd like me.
I'm a nice guy. You'd like me.
Subscribe to:
Posts (Atom)